Integration with SquareSpace

In this tutorial, we’ll aim to go through the necessary steps to connect your Squarespace eCommerce site to a PrinterCo device (TPS900). By doing this, you’ll be able to print receipts for your Squarespace fulfilled orders.
You will need:
- An account with Squarespace (Commerce Advance plan).
- Basic understanding of coding in PHP.
- A server to upload your PHP script.
- Option to set up a Cron Job on your server.
Let’s Get Started!
STEP 1 – Create a Squarespace account.
- If you haven’t already done so, you’ll need to create an account with Squarespace before you begin.
- Click here to jump straight to the sign-up page.
- To get access to the Squarespace API you need to sign-up for the ‘Commerce Advance‘ plan. This will allow you to read the order and transactional data. Click here for Squarespace pricing.

STEP 1 – Create a Squarespace account.
STEP 2 – Create your site.
- Once you’ve logged into your Squarespace account, click the “Create a site” button.
- Provide some optional data to Squarespace about your website, or you can skip this step.
- Select a design.
- Enter your site name and begin customizing your site. You can also skip this step and come back to it later on.
STEP 3 – Generate an API key.
- Now you’ll see the left navigation.
- To generate the API key, click Settings > Advanced > Developer API Keys > Generate Keys.
- Enter a name for this key to help you recognize what this access is for.
- Grant read-only permissions for ‘Orders‘ and ‘Transactions‘.
- Click ‘Generate Key‘ at the top of the pop-up window.
- Copy the API key once it is generated and keep it safe. You won’t be able to view this again.
- Close the pop-up window.
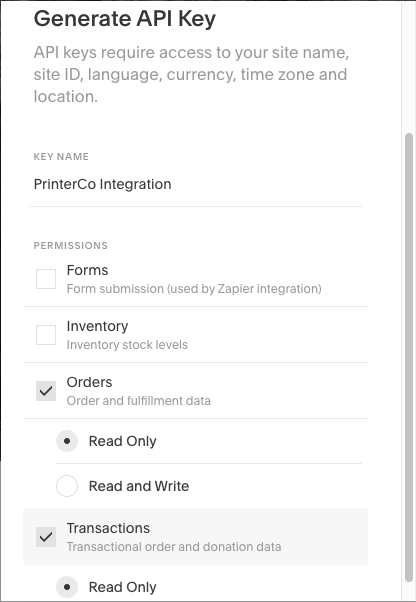
STEP 3 – Generate an API key.
STEP 4 – Write your SquareSpace integration code.
- Copy the PHP template below, making any changes as required.
- If you wish to refer to the Squarespace API documentation, there are 2 API’s you need to focus on.
- Make sure to edit lines 168 and 169 with your own API key and password.
- Also, edit line 177 with your own printer ID
- Upload the PHP file to your web hosting. This script will need to be executed every time a new order is placed on your Squarespace website. We recommend setting up a Cron-Job on your server for this.
<?php
ini_set('display_errors',1);
// ==========================================
// PART 1. Pull order details from SquareSpace
// ==========================================
// Connect to SquareSpace Orders API
$curl_order = curl_init();
curl_setopt_array($curl_order, array(
CURLOPT_URL => "https://api.squarespace.com/1.0/commerce/orders",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_HTTPHEADER => array(
"Authorization: Bearer 5b63af99-9603-42c2-aa80-7600924aa513",
"User-Agent: PostmanRuntime/7.20.1",
),
));
// Connect to SquareSpace Transactions API
$curl_txn = curl_init();
curl_setopt_array($curl_txn, array(
CURLOPT_URL => "https://api.squarespace.com/1.0/commerce/transactions",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_HTTPHEADER => array(
"Authorization: Bearer 5b63af99-9603-42c2-aa80-7600924aa513",
"User-Agent: PostmanRuntime/7.20.1",
),
));
$order_response = curl_exec($curl_order);
$txn_response = curl_exec($curl_txn);
$err_order = curl_error($curl_order);
$err_txn = curl_error($curl_txn);
curl_close($curl_order);
curl_close($curl_txn);
if ($err_order)
{
echo "cURL Error #:" . $err_order;
}
else if ($err_txn)
{
echo "cURL Error #:" . $err_txn;
}
else
{
$order_details = json_decode($order_response, true);
$txn_details = json_decode($txn_response, true);
$order = $order_details["result"][0];
$txn = $txn_details["documents"][0];
// Assign
//echo '<pre>';
//print_r($txn_details["documents"][0]['payments'][0]['id']);
//print_r($txn);
//echo '</pre>';
}
// =========================================================
// PART 2. Set-up required variables from a SquareSpace order - You can find more details for the post fields in your MyPanel dashboard. Visit mypanel.printerco.net
// =========================================================
$order_id = $order['orderNumber'];
$currency = $order['grandTotal']['currency'];
$order_type = 'delivery'; // Only delivery option available in SquareSpace, so static value.
$payment_method = $txn['payments'][0]['provider'];
$auth_code = ''; // Not available in SquareSpace API
$order_time = $order['createdOn'];
$delivery_time = $order['modifiedOn'];
$deliverycost = $order['shippingTotal']['value'];
$card_fee = $txn['payments'][0]['processingFees'][0]['amount']['value'];
$extra_fee = 0.00;
$total_discount = $order['discountTotal']['value'];
$total_amount = $order['grandTotal']['value'];
$cust_name = $order['shippingAddress']['firstName'].' '.$order['shippingAddress']['lastName'];
$cust_address = $order['shippingAddress']['address1'] . "<br>" .
$order['shippingAddress']['address2'] . "<br>" .
$order['shippingAddress']['city'] . "<br>" .
$order['shippingAddress']['state'] . "<br>" .
$order['shippingAddress']['postalCode'] . "<br>";;
if($cust_address==''){
$cust_address = 'Address Not Entered';
}
if($order['shippingAddress']['phone'] == '') {
$cust_phone = 0;
} else {
$cust_phone = $order['shippingAddress']['phone'];
}
$isVarified = 'verified';
$cust_instruction = ''; // Not available in SquareSpace API
$num_prev_order = '';
// =================================================
// Part 3. Save the ordered food items into an array
// =================================================
$str='';
$menu_item = array();
$itemsFinding = $order['lineItems'];
foreach($itemsFinding as $o){
$name = $o['productName'];
$price = $o['unitPricePaid']['value'];
$quantity = $o['quantity'];
// 1.
// Find Product Variants (Size, Colour, etc)
$variants = array();
if(!empty($o['variantOptions'])) {
foreach($o['variantOptions'] as $vo) {
$variants[] = $vo['value'];
}
}
if(!empty($variants)) {
$category = "/r" . implode("/r", $variants);
}
// 2.
// Find form Submissions For Product (Toppings etc)
$form = array();
if(!empty($o['customizations'])) {
foreach($o['customizations'] as $c) {
$form[] = $c['value'];
}
}
if(!empty($form)) {
$option = "/r" . implode("/r", $form);
}
$menu_item[] = array(
'name'=>$name,
'category'=>$category,
'description'=>$option,
'quantity'=>$quantity,
'price'=>$price
);
}
// =============================
// PART 4. API access credential - Find this in your MyPanel dashboard under My account > Edit Information.
// =============================
$api_key = '34c453';
$api_password = '123456';
// ============================
// PART 5. PrinterCo printer ID - visit your MyPanel dashboard and then the Printer List page to find the printer ID.
// ============================
$printer_id = 4481;
// =====================================
// PART 6. Set receipt header and footer
// =====================================
$receipt_header = "PrinterCo Restaurant%%Kemp House, 160 City Road%%London, EC1V 2NX%%0800 689 5326";
$receipt_footer = "Thank you";
// ==========================
// PART 7. Notification URL - When an order is either accepted or rejected on the printer, this info will be sent to this URL.
// ==========================
$notify_url = 'http://yourwebsite.com/notify.php';
// Even if this will not be used, this is a mandatory field needed to send orders to the API
// =========================================
// PART 8. Preparing post fields as an array
// before sending to the PrinterCo API
// =========================================
$post_array = array();
$post_array['api_key'] = $api_key;
$post_array['api_password'] = $api_password;
$post_array['receipt_header'] = $receipt_header;
$post_array['receipt_footer'] = $receipt_footer;
$post_array['notify_url'] = $notify_url;
$post_array['printer_id'] = $printer_id;
$post_array['order_id'] = $order_id;
$post_array['currency'] = $currency;
//1=Delivery, 2=Collection/Pickup, 3=Reservation
if($order_type=='delivery'){
$post_array['order_type'] = 1;
}
else if($order_type=='collection' || $order_type=='pickup'){
$post_array['order_type'] = 2;
}
else if($order_type=='reservation'){
$post_array['order_type'] = 3;
}
//6=Order Paid, 7=Order Not paid
if($payment_method=='STRIPE'){
$post_array['payment_status'] = 6;
}
else{
$post_array['payment_status'] = 7;
} // Add other payment methods as required
$post_array['payment_method'] = $payment_method;
$post_array['auth_code'] = $auth_code;
$post_array['order_time'] = $order_time;
$post_array['delivery_time'] = $delivery_time;
$post_array['deliverycost'] = $deliverycost;
$post_array['card_fee'] = $card_fee;
$post_array['extra_fee'] = $extra_fee;
$post_array['total_discount'] = $total_discount;
$post_array['total_amount'] = $total_amount;
$post_array['cust_name'] = $cust_name;
$post_array['cust_address'] = $cust_address;
$post_array['cust_phone'] = $cust_phone;
//4=verified, 5=not verified
$post_array['isVarified'] = 4;
$post_array['cust_instruction'] = $cust_instruction;
$post_array['num_prev_order'] = $num_prev_order;
$cnt = 1;
foreach($menu_item as $val){
$post_array['cat_'.$cnt] = empty($val['category']) ? "-" : $val['category'];
$post_array['item_'.$cnt] = $val['name'];
$post_array['desc_'.$cnt] = $val['description'];
$post_array['qnt_'.$cnt] = $val['quantity'];
$post_array['price_'.$cnt] = $val['price'];
$cnt++;
}
// ========================================
// Part 8. Post order data to PrinterCo API
// ========================================
$printerco_api_url = 'https://mypanel.printerco.net/submitorder.php';
$response = post_to_api($printerco_api_url,$post_array);
// ===================================
// Part 9. Post data to API using CURL
// ===================================
function post_to_api($url, $post_data) {
set_time_limit(60);
$output = array();
$fields = "";
$i = 0;
foreach ($post_data as $key => $value) {
$fields .= ($i > 0) ? "&" . "$key=" . urlencode($value) : "$key=" . urlencode($value);
$i++;
};
$curlSession = curl_init();
curl_setopt($curlSession, CURLOPT_URL, $url);
curl_setopt($curlSession, CURLOPT_HEADER, 0);
curl_setopt($curlSession, CURLOPT_POST, 1);
curl_setopt($curlSession, CURLOPT_POSTFIELDS, $fields);
curl_setopt($curlSession, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($curlSession, CURLOPT_TIMEOUT, 30);
curl_setopt($curlSession, CURLOPT_SSL_VERIFYPEER, FALSE);
curl_setopt($curlSession, CURLOPT_SSL_VERIFYHOST, 2);
$rawresponse = curl_exec($curlSession);
curl_close($curlSession);
return $output;
}
?>
NOTE:
At the time of this tutorial, Squarespace does not provide a clear distinction between collection and delivery orders. Therefore, we have fixed the variable $order_type as delivery. This variable is mandatory for the PrinterCo API. We recommend getting in touch with Squarespace to see if they have had any updates with their API.
Your Squarespace online ordering system is now ready!
After you’ve configured these settings, your Squarespace website should be ready to print orders. Send a test order from your website and check to see if it is received by your receipt printer. If not, then make sure to check the WatchDog to see if you’ve missed anything.
If you’d like to change the text size and style of the receipt, then make sure you read our tutorial here.
If you need help with your Squarespace receipt printer then feel free to contact our support team. You can email support@printerco.net or reach us via live chat.